The Local Home SDK enhances your smart home integration with Google Assistant by adding a local fulfillment path to route smart home intents.
The Local Home SDK provides two capabilities: Local Execution and Local Query.
- Local Execution provides the ability to fulfill commands by sending
EXEC
intents over the local fulfillment path, instead of making a cloud API call. For example, the request 'Turn on the light' could be handled by Local Execution. - Local Query provides the ability to handle queries concerning
device state by sending
QUERY
intents over the local fulfillment path. For example, Local Query would fulfill the query 'Is my light on?' without making a cloud API call.
The SDK lets you write a local fulfillment app, using TypeScript or JavaScript, that contains your smart home business logic. Google Home or Google Nest devices can load and run your app on-device. Your app communicates directly with your existing smart devices over Wi-Fi on a local area network (LAN) to fulfill user commands, over existing protocols.
Integration of the SDK offers performance improvements to your Cloud-to-cloud integration, including lower latency and higher reliability. Local fulfillment is supported for all device types and device traits, except those that use secondary user verification.
Understand how it works
After getting a SYNC
response
from your cloud fulfillment, the Local Home platform scans the user’s
local area network using mDNS, UDP broadcast, or UPnP to discover
smart devices connected to Assistant.
The platform sends an IDENTIFY
intent to determine if the device is
locally controllable, by comparing the device ID in the IDENTIFY
response
to those returned by the earlier SYNC
response. If the detected device is a
hub or bridge, the platform sends a REACHABLE_DEVICES
intent and treats the
hub as the proxy device for communicating locally.
When it receives a response confirming a local device, the platform establishes a local fulfillment path to the user’s Google Home or Google Nest device, and subsequently routes user commands for local fulfillment.
When a user triggers a Cloud-to-cloud integration that has a local
fulfillment path, Assistant sends the
EXECUTE
intent or
QUERY
intent to the Google Home or Google Nest device
rather than the cloud fulfillment. The device then runs the local fulfillment app to
process the intent.
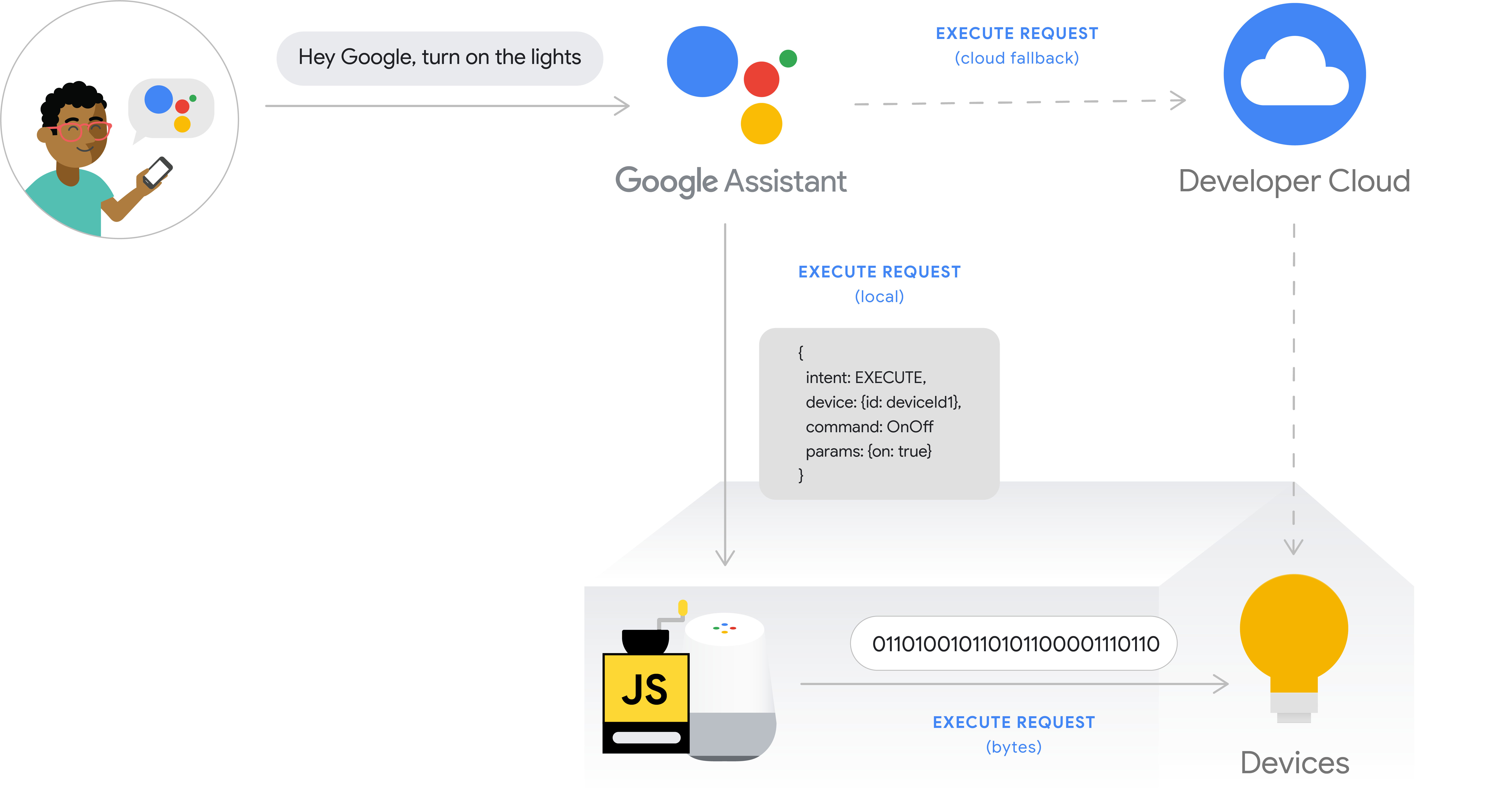
The Local Home platform is non-deterministic in choosing which Google Home or Google Nest device to
trigger the command. The EXECUTE
and QUERY
intents may come through any
Google Home or Google Nest device defined in the same Home Graph
structure as the target device.
Local fulfillment app
The local fulfillment app contains the business logic to process the intents sent by the Local Home platform and access your smart device via the local area network. No modification of your hardware is expected to integrate local fulfillment. The app fulfills Cloud-to-cloud integration requests by sending control commands to the smart device through application-layer protocols, including HTTP, TCP, or UDP. In the event that the local fulfillment path fails, your cloud fulfilment is used as a fallback execution path.
To support building the app, the Local Home SDK provides these two main classes:
DeviceManager
: Provides methods to communicate with smart devices using TCP, UDP sockets, or HTTP requests.App
: Provides methods to attach handlers for intents that Google sends after discovering locally-controllable devices (IDENTIFY
,REACHABLE_DEVICES
). This class also fulfills user commands (EXECUTE
), and answers user queries on current device state (QUERY
).
During development, you can build and test the app by loading it on your own hosting server or local development machine. In production, Google hosts your app in a secure JavaScript sandbox environment on the user’s Google Home or Google Nest device.
The Report State API is not currently supported for local fulfillment. Google relies on your cloud fulfillment to process these requests.
Application lifecycle
Your local fulfillment app is loaded on demand when the Local Home platform discovers new local devices matching the project scan configuration, or has pending intents to deliver related to a previously identified device.
Google Home or Google Nest devices are memory constrained and your local fulfillment app may be terminated at any time due to memory pressure in the system. This can happen if your app starts to consume too much memory, or if the system needs to make room for another app. The Local Home platform restarts your app only when there are new intents to deliver and sufficient memory resources for the app to run.
The Local Home platform unloads your app after an idle timeout when the user
unlinks their account or there are no longer devices that support local
fulfillment associated with the user's agentUserId
.
Supported devices
The Local Home platform executes your local fulfillment app on supported Google Home or Google Nest devices. The following table describes the supported devices, and the runtime used on each device. To learn more about runtime requirements, see Execution environment.
Device | Type | Environment |
---|---|---|
Google Home | Speaker | Chrome |
Google Home Mini | Speaker | Chrome |
Google Home Max | Speaker | Chrome |
Nest Mini | Speaker | Chrome |
Nest Hub | Display | Chrome |
Nest Hub Max | Display | Chrome |
Nest Wifi | Router | Node.js |
Point | Chrome |
Execution environment
The execution environment for your local fulfillment app depends on your device. The Local Home platform supports the following runtime environments:
- Chrome: Your local fulfillment app is executed within the context of a Chrome
browser
window
running Chrome M80 or later with support for ECMAScript version ES2018. - Node.js: Your local fulfillment app is executed as a script within a Node.js process running Node v10.x LTS or later with support for ECMAScript version ES2018.
Source code structure
We recommend bundling your dependencies into a single JavaScript file using the bundler configurations provided by the Local Home SDK and packaging your source code as an Immediately-invoked Function Expression (IIFE).
Implementation path
To utilize the Local Home SDK for your smart home integration, you need to perform these tasks:
1 | Set up the scan config | Configure the Google Home Developer Console with the necessary parameters for the Assistant to discover locally controllable devices. |
2 | Update the SYNC response in your cloud fulfillment | In your cloud fulfillment, modify the
SYNC
request handler to support the
otherDeviceIds field that the platform uses to establish a
local fulfillment path. In that field, specify the IDs of devices that can be
locally controlled.
|
3 | Implement the local fulfillment app | Use the Local Home SDK to create a JavaScript app to
handle the IDENTIFY ,
EXECUTE , and
QUERY intents. For hub or bridge proxy devices, you should
also handle the REACHABLE_DEVICES intent.
|
4 | Test and debug your app | Test your integration (or self-certify) by using the Google Home Test Suite. |
Before you begin
- Familiarize yourself with the basics of creating a Cloud-to-cloud integration.
- In the Google Home Developer Console, make sure you have an existing smart home project and that account linking is configured.
- Make sure that you are logged in with the same Google account in the Developer Console and in Assistant on your test device.
- You'll need a Node.js environment to write your app. For installing Node.js and npm, Node Version Manager is recommended.
- To work with the latest version of the Local Home SDK, you will need to enroll your test devices into the Cast Preview Program.