ZCL Advanced Platform (ZAP) is a node.js template engine that's based on Matter Clusters.
ZAP provides the following features for Matter apps and SDKs:
- Configure Matter Endpoints, Clusters, Attributes, and other device features from a GUI interface.
- Create templates that automatically generate Data Model definitions, callbacks, and other Matter source code.
- Create and use preconfigured ZAP files to include with your SDKs.
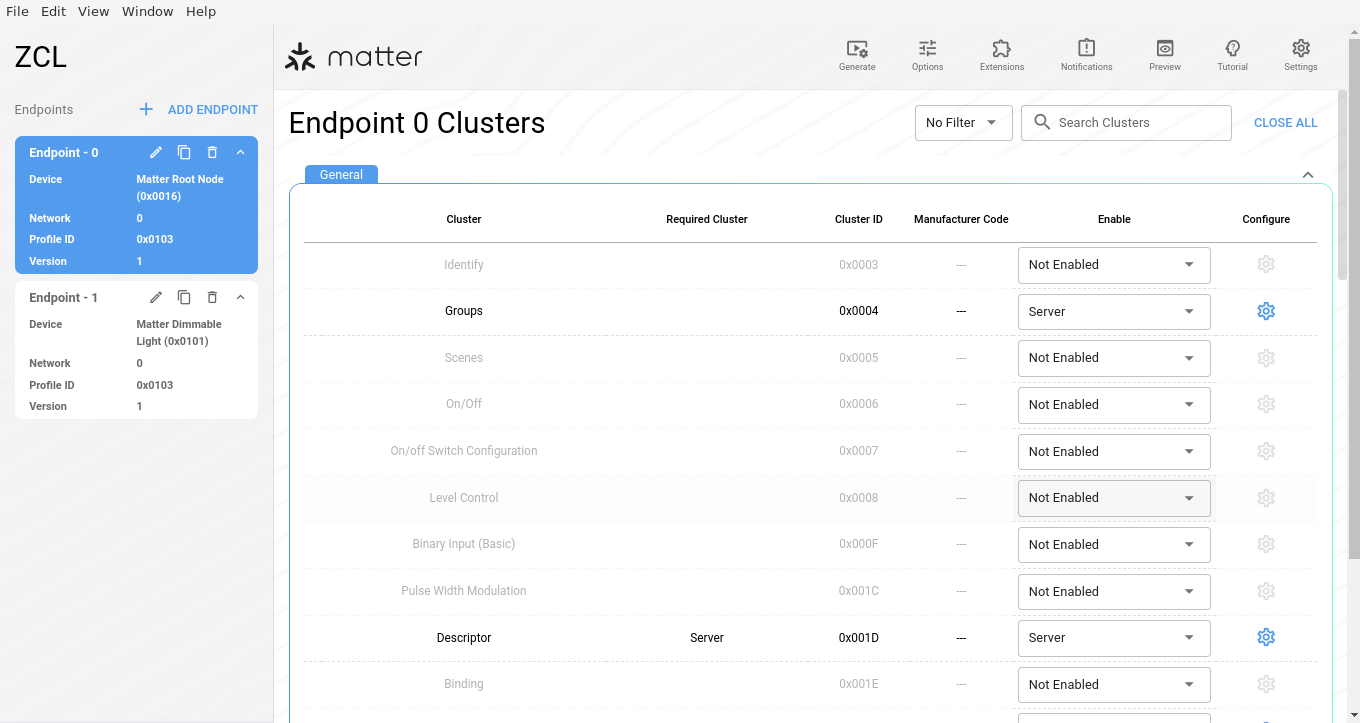
Install
Go to the ZAP releases page.
Look for the release tagged "Latest" (most will be tagged "Pre-release").
Under this release, under the heading Assets, select the package designated for your operating system and download it.
On a machine running Debian or Ubuntu, install ZAP by running:
sudo dpkg -i path/to/zap_installation_package
On Debian or Ubuntu Linux, the zap
binary is installed in /usr/bin/
. On
other operating systems, the binary may be installed elsewhere. In any case,
check to make sure the location of the executable is in your PATH
environment
variable.
ZAP files
ZAP uses template files called ZAP files. A ZAP file is a JSON file
that defines Endpoints, Commands, Attributes, and other device
features. ZAP files have names ending in .zap
. For example,
lighting-app.zap
in connectedhomeip/examples/lighting-app/lighting-common
.
The all-clusters-app.zap
file, found in
connectedhomeip/examples/all-clusters-app/all-clusters-common
, is
preconfigured with common Matter Clusters and three
Endpoints, including a Matter Secondary Network
Commissioning Device Type. This is a good example for exploring various Cluster
configurations.
Run
- Choose a ZAP file from one of the Matter examples.
- From the root directory of the Matter repository (
connectedhomeip
), run therun_zaptool.sh
wrapper script, passing it the path to the.zap
file.
For example:
./scripts/tools/zap/run_zaptool.sh ./examples/lighting-app/lighting-common/lighting-app.zap
Set up
In
Settings you can configure the ZAP user interface, including your choice of light or dark theme, and enabling or disabling the developer tools.Clicking
Options lets you select your Product Manufacturer, Default Response Policy, and enable or disable Command Discovery.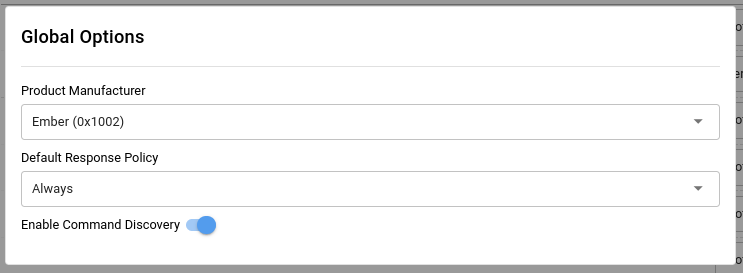
Click
Extensions to add custom ZCL clusters or commands to the Zigbee Clusters Configurator.Use
Endpoints
The lighting-app.zap
file is preconfigured with a Matter
Root Node (Endpoint - 0) and a Matter Dimmable Light
(Endpoint - 1).
Endpoint - 0 includes general Clusters that are relevant to the entire node, for example Networking, Commissioning, Descriptor, Operational Credentials, and OTA Clusters.
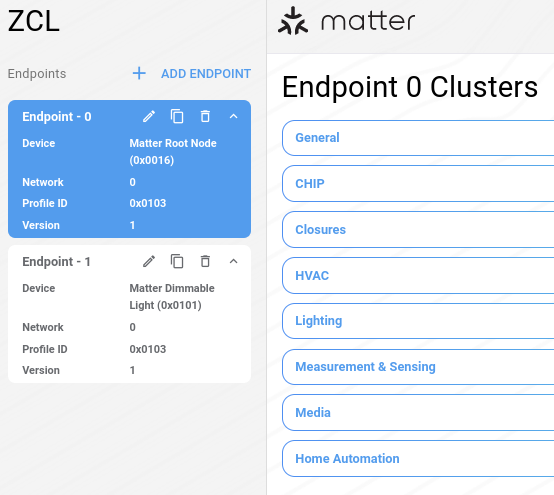
In the next steps, you'll review and configure Endpoint - 1
Matter Dimmable Light from the example lighting-app
.
From the Matter repo
connectedhomeip
, run ZAP.scripts/tools/zap/run_zaptool.sh \ examples/lighting-app/lighting-common/lighting-app.zap
Click Endpoint - 1, then
EDIT.Optional: Matter supports various device types. In the Device dropdown, start typing
matter
to change your device, then click SAVE.
To learn more, refer to Devices and Endpoints in the Matter Primer.
Clusters
Predefined Clusters, Attributes, and Reporting options are enabled in Endpoint - 1 by default.
To display only enabled Clusters, click Endpoint - 1 and select
Enabled Clusters from the Show
menu. You can also search on
.
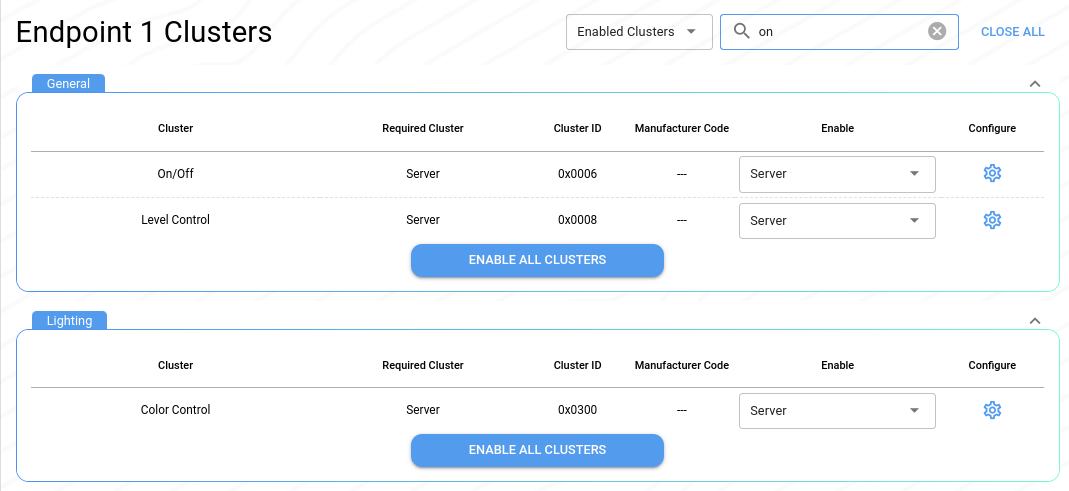
To enable any Cluster as Client, Server or Client & Server, use the Enable dropdown. You can also select Not Enabled to disable a preconfigured Cluster that doesn't apply to your device type.
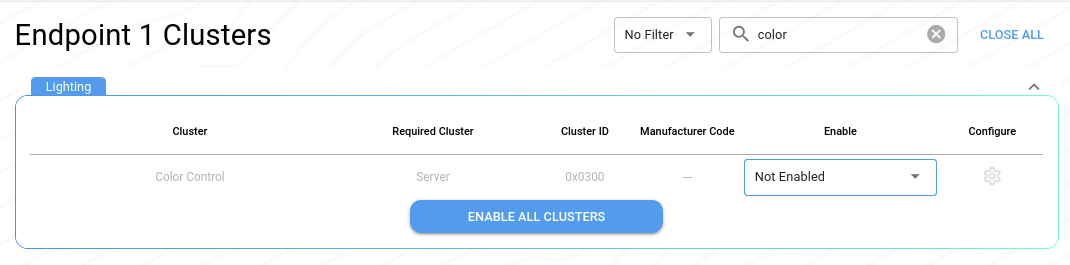
To learn more, refer to Clusters in the Matter Primer.
Attributes and Commands
To configure Attributes and Commands, complete the following steps:
- Locate the
On/Off
Cluster. On the same row, click the Configure icon
.
Here you can enable or disable Attributes, set various Attribute options, and Search attributes
by name.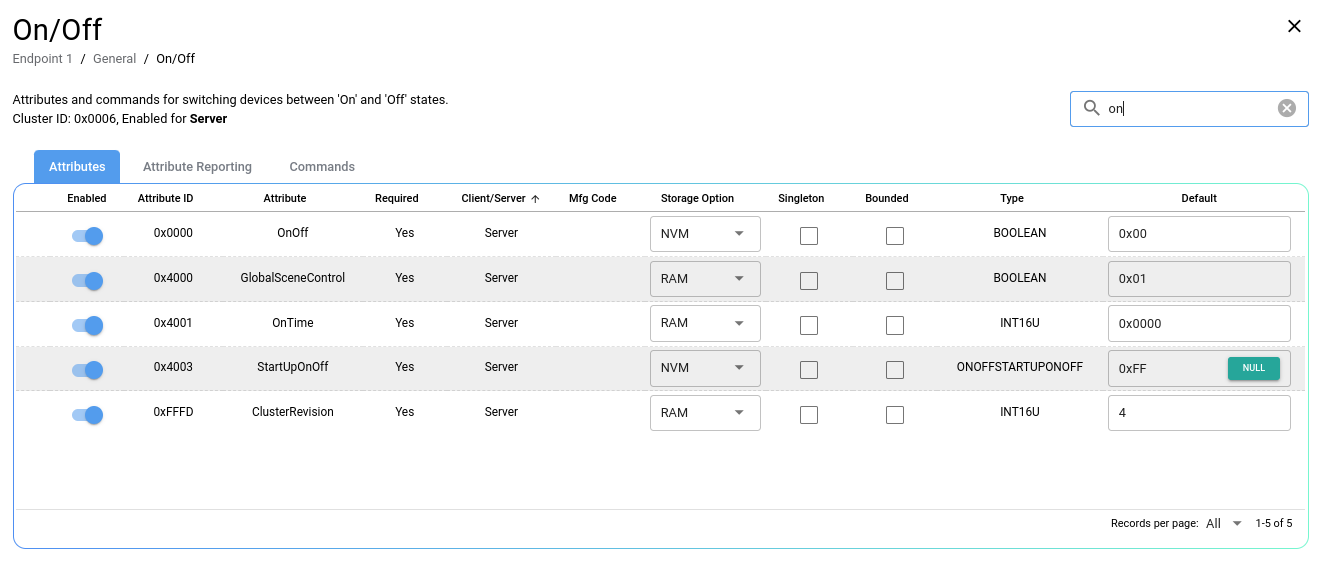
Click Commands to configure actions for this Cluster. For
example, the On/off Cluster for a light will include On, Off,
and Toggle commands. Some Clusters, like
the Temperature Measurement
Cluster, might not have any associated Commands.
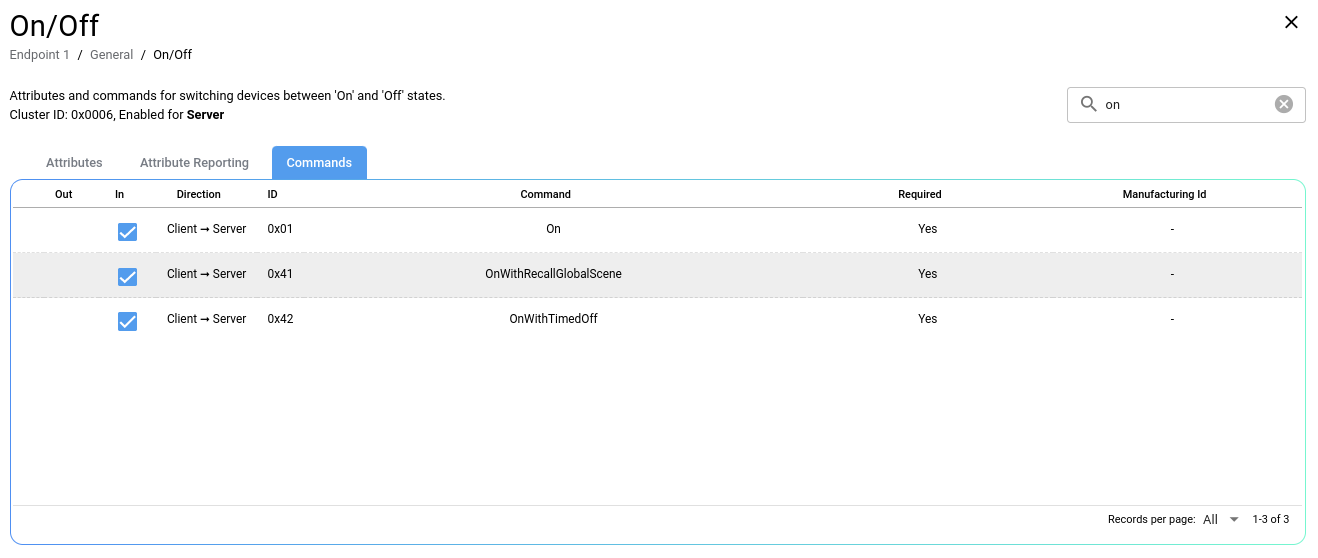
To learn more, refer to Attributes and Commands in the Matter Primer.
Generate ZAP source files
Matter examples use ZAP generated source code, available
in the connectedhomeip/zzz_generated
directory. Some files are app-specific,
while other generic ZAP files are grouped in app-common
.
#include <app-common/zap-generated/ids/Attributes.h>
#include <app-common/zap-generated/ids/Clusters.h>
Once your Endpoints are configured, you can generate source files to integrate into your project.
- Click Generate.
- Select a folder to save the ZAP output. For example, navigate to
connectedhomeip/zzz_generated/lighting-app/zap-generated
for thelighting-app
. - Optional. Open the files in your preferred IDE.
ZAP generates defines, functions, and other source code that's custom to your device type and ZAP configuration.
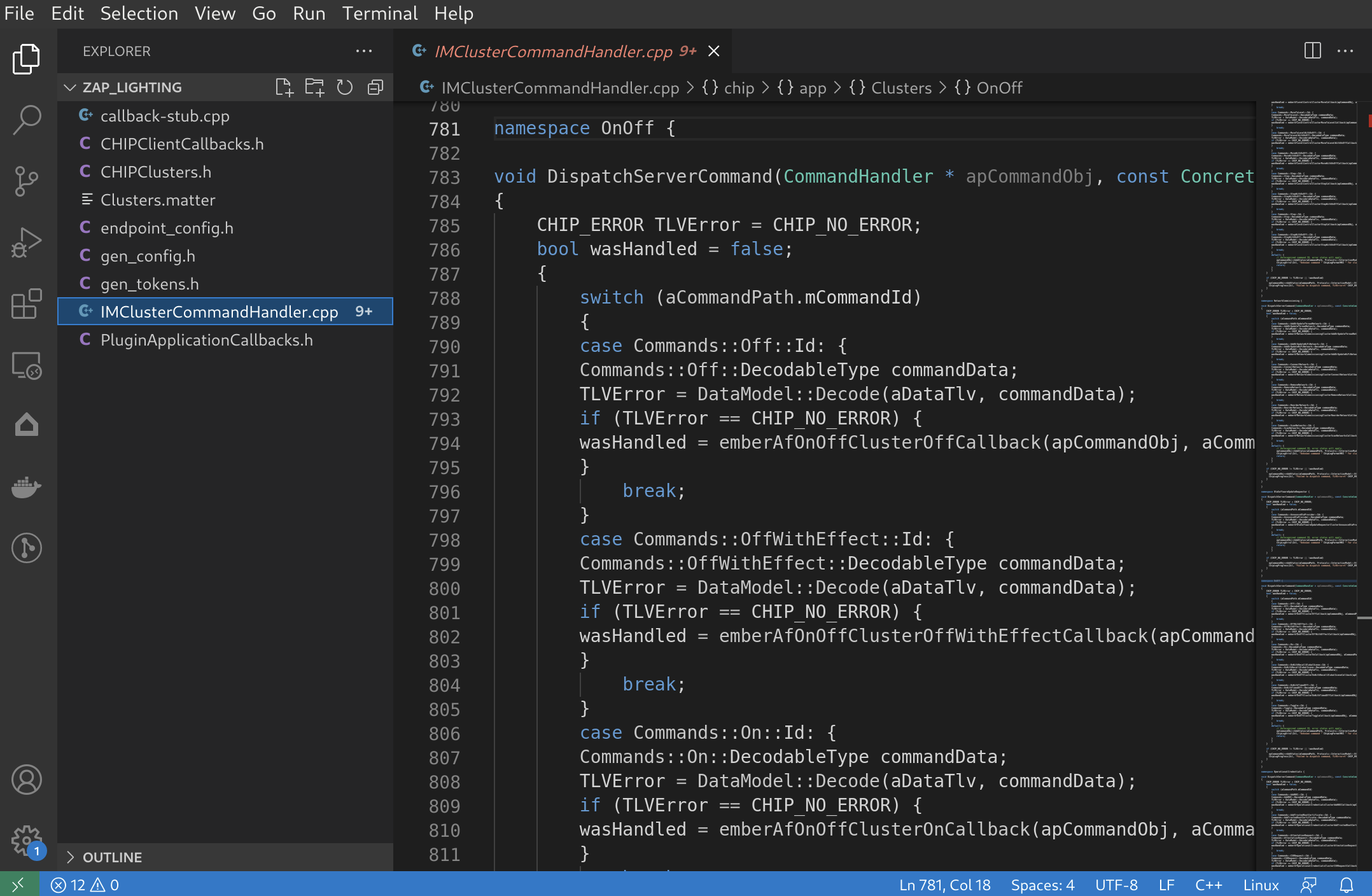
For example, callback-stub.cpp
includes a Cluster callback method that
includes only the Cluster's that you've enabled:
void emberAfClusterInitCallback(EndpointId endpoint, ClusterId clusterId)
{
switch (clusterId)
{
...
case ZCL_ON_OFF_CLUSTER_ID :
emberAfOnOffClusterInitCallback(endpoint);
break;
...
}
}
Use ZAP source files
Common ZAP source files, for example Attributes.h
in
zzz_generated/app-common/app-common/zap-generated/ids/
, can be used to
get and set device type Attributes:
Attributes.h
namespace DeviceTemperatureConfiguration {
namespace Attributes {
namespace CurrentTemperature {
static constexpr AttributeId Id = 0x00000000;
} // namespace CurrentTemperature
...
} // namespace Attributes
} // namespace DeviceTemperatureConfiguration
main.cpp
#include <app-common/zap-generated/att-storage.h>
#include <app-common/zap-generated/attribute-id.h>
#include <app-common/zap-generated/attribute-type.h>
#include <app-common/zap-generated/attributes/Accessors.h>
#include <app-common/zap-generated/callback.h>
#include <app-common/zap-generated/cluster-id.h>
#include <app-common/zap-generated/cluster-objects.h>
#include <app-common/zap-generated/command-id.h>
using namespace ::chip;
using namespace ::chip::app::Clusters;
static void InitServer(intptr_t context)
{
...
// Sets temperature to 20C
int16_t temperature = 2000;
auto status = DeviceTemperatureConfiguration::Attributes::CurrentTemperature::Set(1, temperature);
if (status != EMBER_ZCL_STATUS_SUCCESS)
{
ChipLogError(Shell, "Temp measurement set failed");
}
}
Once you understand how ZAP files can be used in Matter source code, there are several ways that you can integrate ZAP:
- Use the existing
zzz_generated
files to create new examples, unique to your device types. - Create custom
.zap
files for your projects.
Customize
ZAP files are generated from ZAP
templates.
When you use run_zaptool.sh
to launch ZAP, this script passes in the following
templates:
node src-script/zap-start.js --logToStdout \
--gen "$CHIP_ROOT/src/app/zap-templates/app-templates.json" \
--zcl "$CHIP_ROOT/src/app/zap-templates/zcl/zcl.json" \
"${ZAP_ARGS[@]}"
templates.json
defines the Matter template name and
corresponding output file used in Matter examples.
{
"path": "templates/app/callback-stub-src.zapt",
"name": "ZCL callback-stub source",
"output": "callback-stub.cpp"
}
To customize your Matter solutions, there are several next steps that you can take when working with ZAP:
- Create ZAP templates.
- Integrate ZAP into your SDK.
Resources
For additional ZAP resources, refer to the
ZAP repo
(zap
)
on GitHub.