The Commissioning API allows an app to commission to either:
- Your fabric and the Google fabric.
- Only the Google fabric.
Indicate support for Matter commissioning
If you are using the Google Home Mobile SDK to commission, you must
add the app package name
in the Google Home Developer Console, implement our Matter
APIs, and indicate that your app supports Matter
commissioning by handling the ACTION_COMMISSION_DEVICE
intent.
Add the following intent-filter
to the application
declaration within your
AndroidManifest.xml
file:
<intent-filter>
<action android:name="com.google.android.gms.metadata.MODULE_DEPENDENCIES" />
</intent-filter>
See our sample app's manifest for reference.
Ways to commission Matter devices
The commissioning process can be initiated:
Request commissioning directly in your app
Requesting commissioning directly in the app can be triggered by a button in the app, and can be done in two ways:
For single fabrics
To request commissioning:
Initialize an
ActivityResultLauncher
in your activity. If the user commissioned the device on the Google fabric, the result may include the name the user assigned to the device when they commissioned it.private val commissioningLauncher = registerForActivityResult(StartIntentSenderForResult()) { result -> val resultCode = result.resultCode if (resultCode == RESULT_OK) { Log.i("CommissioningActivity", "Commissioning success") val deviceName = CommissioningResult.fromIntentSenderResult(result.resultCode, result.data).deviceName } else { Log.i("CommissioningActivity", "Commissioning failed") } }
Construct a
CommissioningRequest
, including the received payload data, and set the option to commission the device to the Google fabric, usingsetStoreToGoogleFabric
:val commissioningRequest = CommissioningRequest.builder() .setOnboardingPayload(payload) .setStoreToGoogleFabric(true) // set all other options that you care about .build()
If you want to commission the device to the Google fabric as well as your own fabric, set your commissioning service with
setCommissioningService
in theCommissioningRequest
.Use the
CommissioningClient
instance to start commissioning:commissioningClient .commissionDevice(commissioningRequest) .addOnSuccessListener { result -> Log.i("CommissioningActivity", "Commissioning success") _commissioningIntentSender.postValue(result) } .addOnFailureListener { error -> Log.i("CommissioningActivity", "Commissioning failed") }
Where
_commissioningIntentSender
is defined as:private val _commissioningIntentSender = MutableLiveData<IntentSender?>() val commissioningIntentSender: LiveData<IntentSender?> get() = _commissioningIntentSender
Once the
CommissioningClient
returns the intent sender, launch the sender:commissioningIntentSender.observe(this) { sender -> if (sender != null) { commissioningLauncher.launch(IntentSenderRequest.Builder(sender).build()) } }
For multiple fabrics (multi-admin)
If you need to set up multiple Matter fabrics on a device, refer to How to use commissioning APIs in multi-admin mode.
Matter commissioning entry point for Fast Pair or QR-code scanning (Android only)
Requesting commissioning by means of fast pair or QR code on Android can be done in two ways:
For single fabrics
Use the ACTION_START_COMMISSIONING
intent filter
to provide full commissioning capability for an app without needing
the GHA. When commissioning to the Google fabric, this
includes allowing the user to assign a name to the device.
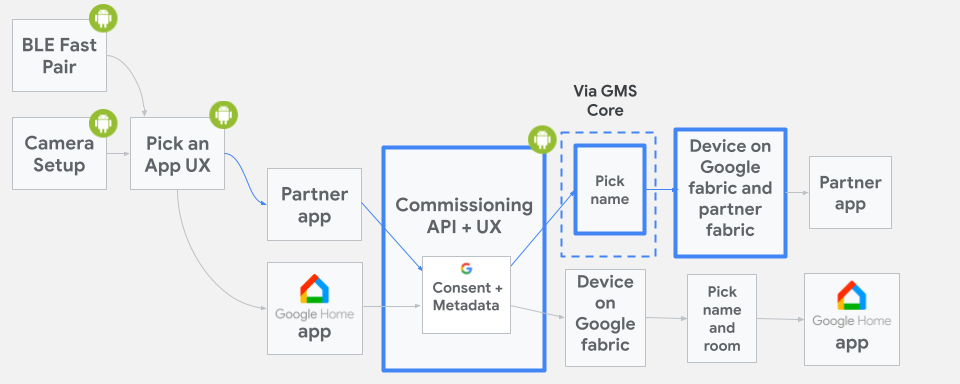
ACTION_START_COMMISSIONING
To indicate support for Google fabric commissioning, add the following
intent-filter
to the chosen activity declaration within your
AndroidManifest.xml
file:
<intent-filter>
<action android:name="com.google.android.gms.home.matter.ACTION_START_COMMISSIONING" />
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
The intent-filter
is used to include your app in the list of suggested
Matter apps in the Commissioning APIs app
picker.
If your app is not one of the suggested apps, it appears in the Choose other
app option.
Once the user selects your app, your app is launched and directed to the chosen
activity with an
ACTION_START_COMMISSIONING
intent.
For multiple fabrics (multi-admin)
you can also use the FastPair flow in multi-admin scenarios. For more information, refer to How to use commissioning APIs in multi-admin mode.
Handle the incoming intent
Once your activity is launched, it should check for the existing
ACTION_START_COMMISSIONING
intent and retrieve the payload:
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
val payload = if (Matter.ACTION_START_COMMISSIONING.equals(intent.getAction())) {
intent.getStringExtra(Matter.EXTRA_ONBOARDING_PAYLOAD)
} else {
null
}
CommissioningRequest.builder()
.setOnboardingPayload(payload)
.setStoreToGoogleFabric(true)
// set all other options that you care about
startCommissioning(commissioningRequest)
}
A payload value that is not null
indicates that the user has already either
scanned the device QR code, or entered the pairing key. A null
payload value
does not mean that the commissioning should be aborted.
Suppress commissionable discovery notifications
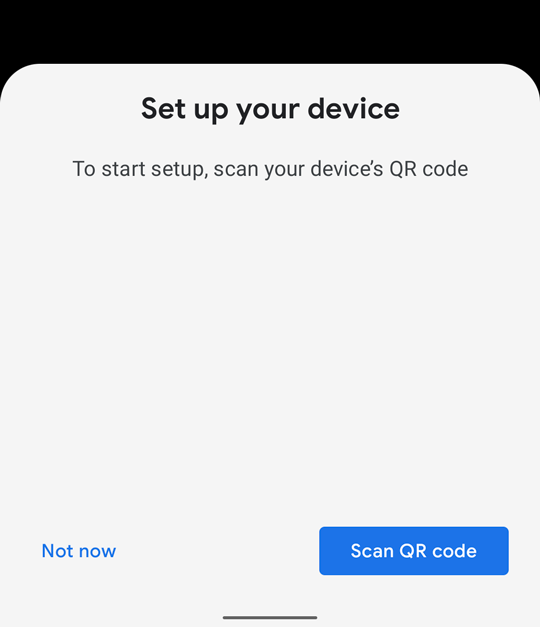
By default, Google Play services on Android uses "halfsheet" notifications that cover the bottom half of a mobile device's screen to provide users with a proactive indication that commissionable Matter devices are nearby.
To prevent interruptions while your app is in the foreground, you may
suppress these notifications by calling the
suppressHalfSheetNotification()
method in the Mobile SDK. See the API documentation for more
information.
The suppression enabled by this API times out if your app is in the foreground
for more than 15 minutes. To re-enable suppression after a timeout, call
suppressHalfSheetNotification()
again, otherwise halfsheet notifications will
start to appear.
An implementation of this API can be found in the
Google Home Sample App for Matter. See
HalfSheetSuppressionObserver.kt
for more information.
How should you share Matter devices on your fabric with Google?
Google strongly recommends that you use the Commissioning API as your primary means of sharing a device that you have already set up on your own fabric with the Google fabric. The Share API has its limitations and should be reserved for other use-cases.
Why should you use the Commissioning API instead of the Share API?
The Commissioning API lets you trigger sharing a device directly with the Google fabric, which is the preferred method when viable. With the Share API, more steps are required for the end user. For example, the end user must have GHA installed, and they must know to select GHA during the process to ensure success.
To use the Commissioning API, you should open the commissioning window and call the Commissioning API, as described in How to use the Commissioning API as the secondary Matter commissioner.
When should you use the Share API?
You can use the Share API to let the end-user pick an eligible application to share a device generically with other Matter ecosystems.